Pages
Thursday, September 29, 2016
t-famil"
Wednesday, September 28, 2016
Add Marker to Google Map Google Maps Android API v2
To add marker to Google Map of Google Maps Android API v2:
LatLng targetLatLng = new LatLng(lat, lon);
MarkerOptions markerOptions =
new MarkerOptions().position(targetLatLng).title(strTitle);
mMap.addMarker(markerOptions);
Modify from last example "Set map type for Google Maps Activity using Google Maps Android API v2" to add feature to add markers on Google Maps Activity.


Edit values/strings.xml to add string resource of "menu_addmarkers"
<resources>
<string name="app_name">AndroidStudioMapApp</string>
<string name="title_activity_maps">Map</string>
<string name="menu_legalnotices">Legal Notices</string>
<string name="menu_about">About</string>
<string name="menu_addmarkers">Add Markers</string>
</resources>
Edit menu/activity_main.xml, to add menu option of Add Markers:
<?xml version="1.0" encoding="utf-8"?>
<menu
>
<item
android_id="@+id/menu_about"
android_orderInCategory="100"
app_showAsAction="ifRoom"
android_title="@string/menu_about"/>
<item
android_id="@+id/menu_addmarkers"
android_orderInCategory="100"
app_showAsAction="ifRoom"
android_title="@string/menu_addmarkers"/>
<item
android_id="@+id/maptype"
android_orderInCategory="100"
app_showAsAction="never"
android_title="Map Type...">
<menu >
<group android_id="@+id/groupmaptype"
android_checkableBehavior="single">
<item android_id="@+id/maptypeNORMAL"
android_title="NORMAL" />
<item android_id="@+id/maptypeSATELLITE"
android_title="SATELLITE" />
<item android_id="@+id/maptypeTERRAIN"
android_title="TERRAIN" />
<item android_id="@+id/maptypeHYBRID"
android_title="HYBRID" />
<item android_id="@+id/maptypeNONE"
android_title="NONE" />
</group>
</menu>
</item>
<item
android_id="@+id/menu_legalnotices"
android_orderInCategory="100"
app_showAsAction="never"
android_title="@string/menu_legalnotices"/>
</menu>
Modify MapsActivity.java
package com.blogspot.android_er.androidstudiomapapp;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.text.InputType;
import android.view.Menu;
import android.view.MenuItem;
import android.widget.EditText;
import android.widget.LinearLayout;
import android.widget.Toast;
import com.google.android.gms.common.GoogleApiAvailability;
import com.google.android.gms.maps.CameraUpdateFactory;
import com.google.android.gms.maps.GoogleMap;
import com.google.android.gms.maps.OnMapReadyCallback;
import com.google.android.gms.maps.SupportMapFragment;
import com.google.android.gms.maps.model.LatLng;
import com.google.android.gms.maps.model.MarkerOptions;
public class MapsActivity extends AppCompatActivity implements OnMapReadyCallback {
private GoogleMap mMap;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_maps);
// Obtain the SupportMapFragment and get notified when the map is ready to be used.
SupportMapFragment mapFragment = (SupportMapFragment) getSupportFragmentManager()
.findFragmentById(R.id.map);
mapFragment.getMapAsync(this);
}
/**
* Manipulates the map once available.
* This callback is triggered when the map is ready to be used.
* This is where we can add markers or lines, add listeners or move the camera. In this case,
* we just add a marker near Sydney, Australia.
* If Google Play services is not installed on the device, the user will be prompted to install
* it inside the SupportMapFragment. This method will only be triggered once the user has
* installed Google Play services and returned to the app.
*/
@Override
public void onMapReady(GoogleMap googleMap) {
mMap = googleMap;
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.activity_main, menu);
return super.onCreateOptionsMenu(menu);
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case R.id.menu_addmarkers:
addMarker();
return true;
case R.id.maptypeHYBRID:
if(mMap != null){
mMap.setMapType(GoogleMap.MAP_TYPE_HYBRID);
return true;
}
case R.id.maptypeNONE:
if(mMap != null){
mMap.setMapType(GoogleMap.MAP_TYPE_NONE);
return true;
}
case R.id.maptypeNORMAL:
if(mMap != null){
mMap.setMapType(GoogleMap.MAP_TYPE_NORMAL);
return true;
}
case R.id.maptypeSATELLITE:
if(mMap != null){
mMap.setMapType(GoogleMap.MAP_TYPE_SATELLITE);
return true;
}
case R.id.maptypeTERRAIN:
if(mMap != null){
mMap.setMapType(GoogleMap.MAP_TYPE_TERRAIN);
return true;
}
case R.id.menu_legalnotices:
String LicenseInfo = GoogleApiAvailability
.getInstance()
.getOpenSourceSoftwareLicenseInfo(MapsActivity.this);
AlertDialog.Builder LicenseDialog =
new AlertDialog.Builder(MapsActivity.this);
LicenseDialog.setTitle("Legal Notices");
LicenseDialog.setMessage(LicenseInfo);
LicenseDialog.show();
return true;
case R.id.menu_about:
AlertDialog.Builder aboutDialogBuilder =
new AlertDialog.Builder(MapsActivity.this);
aboutDialogBuilder.setTitle("About Me")
.setMessage("http://android-er.blogspot.com");
aboutDialogBuilder.setPositiveButton("visit",
new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
String url = "http://android-er.blogspot.com";
Intent i = new Intent(Intent.ACTION_VIEW);
i.setData(Uri.parse(url));
startActivity(i);
}
});
aboutDialogBuilder.setNegativeButton("Dismiss",
new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
dialog.cancel();
}
});
AlertDialog aboutDialog = aboutDialogBuilder.create();
aboutDialog.show();
return true;
}
return super.onOptionsItemSelected(item);
}
private void addMarker(){
if(mMap != null){
//create custom LinearLayout programmatically
LinearLayout layout = new LinearLayout(MapsActivity.this);
layout.setLayoutParams(new LinearLayout.LayoutParams(
LinearLayout.LayoutParams.MATCH_PARENT,
LinearLayout.LayoutParams.WRAP_CONTENT));
layout.setOrientation(LinearLayout.VERTICAL);
final EditText titleField = new EditText(MapsActivity.this);
titleField.setHint("Title");
final EditText latField = new EditText(MapsActivity.this);
latField.setHint("Latitude");
latField.setInputType(InputType.TYPE_CLASS_NUMBER
| InputType.TYPE_NUMBER_FLAG_DECIMAL
| InputType.TYPE_NUMBER_FLAG_SIGNED);
final EditText lonField = new EditText(MapsActivity.this);
lonField.setHint("Longitude");
lonField.setInputType(InputType.TYPE_CLASS_NUMBER
| InputType.TYPE_NUMBER_FLAG_DECIMAL
| InputType.TYPE_NUMBER_FLAG_SIGNED);
layout.addView(titleField);
layout.addView(latField);
layout.addView(lonField);
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setTitle("Add Marker");
builder.setView(layout);
AlertDialog alertDialog = builder.create();
builder.setPositiveButton("OK", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
boolean parsable = true;
Double lat = null, lon = null;
String strLat = latField.getText().toString();
String strLon = lonField.getText().toString();
String strTitle = titleField.getText().toString();
try{
lat = Double.parseDouble(strLat);
}catch (NumberFormatException ex){
parsable = false;
Toast.makeText(MapsActivity.this,
"Latitude does not contain a parsable double",
Toast.LENGTH_LONG).show();
}
try{
lon = Double.parseDouble(strLon);
}catch (NumberFormatException ex){
parsable = false;
Toast.makeText(MapsActivity.this,
"Longitude does not contain a parsable double",
Toast.LENGTH_LONG).show();
}
if(parsable){
LatLng targetLatLng = new LatLng(lat, lon);
MarkerOptions markerOptions =
new MarkerOptions().position(targetLatLng).title(strTitle);
mMap.addMarker(markerOptions);
mMap.moveCamera(CameraUpdateFactory.newLatLng(targetLatLng));
}
}
});
builder.setNegativeButton("Cancel", null);
builder.show();
}else{
Toast.makeText(MapsActivity.this, "Map not ready", Toast.LENGTH_LONG).show();
}
}
}

Next:
- initialize map in xml
~ Step-by-step of Android Google Maps Activity using Google Maps Android API v2, on Android Studio
Haydenshapes helps surfers catch the perfect wave with Google Apps
Editors note: Todays guest blogger is Hayden Cox, founder and owner of Haydenshapes Surfboards and creator of FutureFlex technology. The Haydenshapes team uses Google Apps for Work to stay in touch and get more done across oceans and time zones.
When I was 15 years old, I snapped my favourite board while surfing on Sydneys northern beaches. I didnt have the money to replace it, so I built one instead. I spent my next school vacation volunteering at a local factory, where I learned the basics of shaping and making surfboards. Thats how I first developed a passion for creating and design. After school and on weekends, Id shape boards for friends and teachers, who eventually became repeat customers and recommended me to their friends. It occurred to me that I should formalise what I was doing, so I created a logo and started branding my boards. I also taught myself how to code my first website. Thats how Haydenshapes Surfboards got started.
Fast-forward 18 years, and Haydenshapes has come a long way. We have our own signature surfboard technology, FutureFlex, which I patented and designed. We sell surfboards in 70 countries around the world, from India and Israel to Sri Lanka and Sweden even in places that I didnt realise had surf. Our Hypto Krypto model is the best-selling surfboard worldwide and was named Surfboard of the Year at the 2014 and 2015 Australian Surf Industry Awards.

As weve grown, Ive learned some fundamental realities of running a business. Mistakes are inevitable, so its crucial to keep your finger on the pulse 24/7. Also, its important to keep things simple so you can work smarter rather than harder. Google Apps for Work helps us do that. It provides the full framework we need to run our business and connect our teams and manufacturing centers in Sydney, Los Angeles and Thailand. Its reliable, secure and easy to use and it just works.

Im constantly on the go, whether Im racing off of a plane, jumping out from the surf, in the shaping bay, or between meetings. Being able to tap into our Google Apps system from my device means Im always aware of whats going on. I can share design files with Drive to our CNC machines, draft budgets with Google Sheets, and use Hangouts for meetings and video calls with my team and family.

Haydenshapes is known for being innovative and progressive. Our products and our process how we do things from start to finish reflect this. In my opinion, your success hinges on how you spend your time. The last thing we want to do is worry about servers failing or files getting backed up properly. Working with a seamless system like Google Apps means that I can focus on what really drives the business: designing and creating the best surfboards.
Tuesday, September 27, 2016
HTC One M9 in all flavours! Gallery
...and tell us, whats your favourite colour? :)

























Do you have any questions or comments? Feel free to share! Also, if you like this article, please use media sharing buttons (Twitter, G+, Facebook) below this post!



Sunday, September 25, 2016
Mobile Andrio Full apk

Mobile Andrio Full Overview: A jump & run game in the style of Super Mario, Giana Sisters and similar games.
A jump-and-run game in the style of Super Mario, Giana Sisters and similar games. It has 16 levels of increasing difficulty.
Highscores get uploaded to the social gaming network Scoreloop, and you can compare your highscore with those of players all around the world. Furthermore you can start challenge games against other players to determine the better player in a 1:1 situation.
The game can be controlled using keyboard (preferred), touchscreen, orientation sensor or trackball. Use Edit Settings to customize.
Full version, free of ads. Permissions required for Scoreloop features.
For more information, please check the apps website.
Whats in Mobile Andrio Full :
- improved the placement of the on-screen controls
- bug fixes
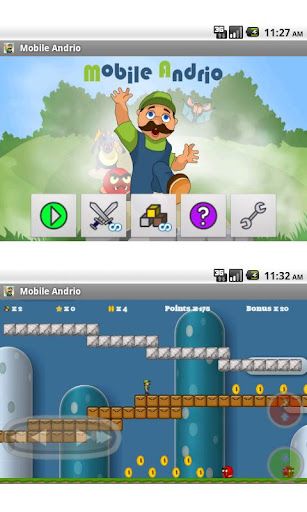
Saturday, September 24, 2016
eBoostr 4 5 Full Cracked Alternate RAM for PC Download Direct!!
eBoostr 4.5 Full Cracked. Alternate RAM for PC Download Direct!!


eBoostr adalah salah satu software unik yang mampu mengubah media penyimpanan data luar seperti flashdisk dan memory card menjadi perangkat RAM. eBoostr tidak dapat menggunakan harddisk sebagai penyedia RAM, hanya 'removable disk' saja. Untuk melihat cara mengubah harddisk menjadi RAM, simak baik-baik artikel ini:
Ubah Flashdisk, HARD DISK/HARD DRIVE, Memory card jadi RAM Komputer
Friday, September 23, 2016
The Total Economic Impact™ of Google Apps for Work
Editors note: Its easy to see how real-time collaboration eliminates frustration from your day or how mobile access from anywhere helps keep your projects moving forward even when youre on the go, but it takes a bit of number crunching to understand the impact across an entire company. To help make this easier, we commissioned Forrester Consulting to quantify the benefits a typical Google Apps for Work customer enjoys.
Companies across the globe face increasing pressure to stay competitive and meet their customers needs. Tools that allow teams to share ideas instantly, attend meetings remotely, collaborate from anywhere in real time and work on the go are helping companies innovate and engage customers in this new competitive landscape. These types of outcomes are possible only by pure cloud-based architectures that overcome the inefficiencies of legacy desktop- centric computing.
While its easy to understand how collaboration and mobility impact our day-to-day work, its more difficult for organizations to quantify these benefits in monetary terms.
So Google commissioned Forrester Consulting to conduct a Total Economic Impact (TEI) study examining the value that Google customers achieve by implementing Google Apps for Work. Forrester measured the total economic impact over three years for organizations moving from legacy on-premise infrastructure to Googles web-based solution. To quantify the complete value of Google Apps for Work, including collaboration and productivity benefits, they interviewed six of our current customers. They then aggregated each piece of customer feedback to create a representative composite organization on which to base the development of a Total Economic Impact model.
The composite organization is a global B2B multinational services company with 10,000 employees using Google Apps for Work and $4 billion in annual revenue. The analysis they completed showed that this composite organization would realize millions in collaboration and mobility efficiencies in the course of three years.
Here are a few highlights from the report:
- 304% return on investment (ROI) Over three years, Google Apps for Work generated a risk-adjusted $17.1 million in benefits, outweighing the total costs of $4.2 million and resulting in a risk-adjusted ROI of 304%.
- $8 million in collaboration efficiencies: Employees can streamline business processes by working together in real -time using Google Docs, Sheets, and Slides, creating project collaboration spaces in Google Sites, and accessing and sharing files with Google Drive. These collaboration efficiencies save employees up to two hours per week which, over three years, adds up to more than $8 million in savings.
- $9 million in mobility benefits and legacy IT cost savings Google Apps for Work creates an environment where employees can work together, share ideas, innovate, evaluate decisions and improve business performance all without having to be in a physical office. The ability to work from anywhere and join meetings remotely saves the composite organization more than $5 million in 3 years, while decommissioning legacy servers, software and phone systems saves another $4 million. And $9 million can go a long way.
Though these findings are the result of in-depth analysis, we strongly encourage any Google Apps customer to conduct their own impact analysis to see what specific benefits they experience from using Google Apps for Work. You can read the full study, The Total Economic Impact of Google Apps for Work, by visiting g.co/AppsEconomicImpact.

Know the news from Google Android

Google just announced:
- New Nexus phone running Marshmallow: Nexus 6P and 5X
- The first Android tablet built by Google, Pixel C, and the Chromebook Pixel
- The new Chromecast and Chromecast Audio
- Google Play Music and Google Photos
Google Press Event 9/29/15
Check Official Google Blog
Cheat Lost Saga 2015 No Delay Kebal Max combo kill Brust Mafia Speed UP UN TOKEN ONE HIT Dugeon CSD
Cheat Lost Saga 2015 ( No Delay, Kebal, Max combo kill, Brust Mafia, Speed UP, UN TOKEN,ONE HIT Dugeon CSD )

Download :
No Password
Pilih Injector diatas ini Sesuka anda Jika Cheat Error Gunakan Injector yang lain.
Hotkey Simple Langsung aktif d3d menu
- No Delay
- Unl Hp
- Kebal
- Max combo kill
- fullhack dll
Fiture SimpleCheat :
Tutorial :
- Buka Cheat Lost Saga
- Tunggu Keluar Notice selesai - > Cheat Aktif
- Buka Lost Saga
- Star Lost Saga
Wednesday, September 21, 2016
App avast! Mobile Security v1 0 1282


avast! Mobile Security berfungsi sebagai proteksi data pribadi sekaligus sebagai antivirus dan melindungi dari website yang berbahaya. Aplikasi ini juga bisa berfungsi sebagai firewall dari tangan hacker. Fungsi-fungsi yang ada misalnya: mengontrol fitur anti-pencurian melalui sms: menghapus memori, mengunci hanphone, mengaktifkan sirine dan GPS, memonitor suara dan masih banyak lagi. Fitur-fitur penting avast! Mobile Security selain disebutkan di atas antara lain:
- Antivirus
- Privacy Report
- SMS/Call Filtering
- App Manager
- Firewall
- avast! Anti-Theft
- App Disguiser
- Stealth Mode
- Self-Protection
- Battery Save
- SIM-Card-Change Notification
- Trusted SIM Cards List
- Remote Settings Change
- Remote Features
Download: filesonic | filepost (1.84 MB)
Tuesday, September 20, 2016
The Lost Treasure v1 3 Apk For Android
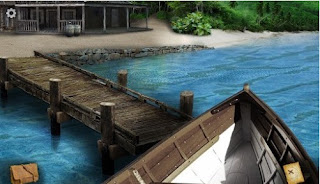
Uncle Henry has been hunting lost treasures for as long as you can remember. His legendary stories of adventure excited your imagination as you were a child growing up. Now with your newly acquired archeology skills, he has been reaching out from time to time for your help in tracking down some of these difficult to find treasures.
In his latest quest, he has used the map you discovered in the lost ship and tracked down the location of the pirates cove. You must explore the island, find clues, and solve puzzles left behind by the pirates to discover the location of the lost treasure!
This captivating adventure game has:
- Custom designed beautiful HD graphics!
- Custom composed soundtrack and sound effects!
- A built-in hint system for when you get stumped
- A dynamic map to show the screens you have visited and current location
- A camera that takes photos of clues and symbols as you discover them
- Dozens of puzzles, clues, and items
- Auto saves your progress
- Available for phones and tablets!
Whats new:
Added immersive full-screen layout for Android
This app has no advertisements
Download APK:
click here
Download DATA:
click here
Google Play Store:
click here
Stock system dumps for HTC One HTC One X

These executable (.exe) files for MS Windows are installers of complete firmware, including for example system or data partitions. Not having latest RUU means:
- users using custom ROMs cant download latest OTA from HTC,
- developers have no system dumps they can work with.
- adb pull /system in custom recovery mode*
- cp /system /data/media/dump in custom recovery mode*
- nandroid backup
- boot partition can be taken from inside firmware.zip inside OTA .zip package or it can be dumped using dd if=</path/to/boot/partition> of=/data/media/boot.img
Sunday, September 18, 2016
Mark the date Google Calendar for iPhone is here
Since the ancient Sumerian calendar that recorded a new month by the sighting of a new moon, calendars both physical and digital have helped us keep track of important moments. Today, were bringing the new Google Calendar app to the iPhone, so you can spend less time managing your day and more time getting stuff done.
The new Google Calendar app for iPhone comes with Assists, which make suggestions that save you time when creating events. For example, if you regularly meet with your teammate Rena for coffee and a catchup, Calendar can quickly suggest that entire event when you type c-o-f-. With Schedule View, a quick glance gives you a snapshot of how your day is shaping up with images and maps that make your calendar easy to scan.
To get started simply login with your Google account, and with one click, the new app works with all the other calendars youve already set up on your iPhone.

And if you end up leaving your phone in the taxi on the way back to the office, Google Apps Mobile Management has you covered. Apps Mobile Management allows admins to set up mobile security policies, like requiring passwords and remotely wiping data when necessary. And its included with Google Apps and Google Drive for Work, so you can keep your work data safe.
10 minutes and counting until your next meeting . . . download the new Google Calendar app for iPhone before it starts.
Building a scalable geofencing API on Google’s App Engine
Googles Cloud Platform offers a great set of tools for creating easily scalable applications in the cloud. In this post, Ill explore some of the special challenges of working with geospatial data in a cloud environment, and how Googles Cloud can help. Ive found that there arent many options to do this, especially when dealing with complicated operations like geofencing with multiple complex polygons. You can find the complete code for my approach on GitHub.
Geofencing is the procedure of identifying if a location lies within a certain fence, e.g. neighborhood boundaries, school attendance zones or even the outline of a shop in a mall. Its particularly useful in mobile applications that need to apply this extra context to someones exact location. This process isnt actually as straight forward as youd hope and, depending on the complexity of your fences, can include some intense calculations and if your app gets a lot of use, you need to make sure this doesnt impact performance.
In order to simplify this problem this blogpost outlines the process of creating a scalable but affordable geofencing API on Googles App Engine.
And the best part? Its completely free to start playing around.
Getting started
To kick things off you can work through the Java Backend API Tutorial. This uses Apache Maven to manage and build the project.If you want to dive right in you can download the finished geofencing API from my GitHub account.
The Architecture
The requirements are:- Storing complex fences (represented as polygons) and some metadata like a name and a description. For this I use Cloud Datastore, a scalable, fully managed, schemaless database for storing non-relational data. You can even use this to store and serve GeoJSON to your frontend.
- Indexing these polygons for fast querying in a spatial index. I use an STR-Tree (part of JTS) which I store as a Java Object in memcache for fast access.
- Serving results to multiple platforms through HTTP requests. To achieve this I use Google Cloud Endpoints, a set of tools and libraries that allow you to generate APIs and client libraries.
Thats all you need to get started - so lets start cooking!
Creating the Project
To set up the project simply use Apache Maven and follow the instructions here. This creates the correct folder structure, sets up the routing in the web.xml file for use with Googles API Explorer and creates a Java file with a sample endpoint.Adding additional Libraries
Im using the Java Topology Suite to find out which polygon a certain latitude-longitude-pair is in. JTS is an open source library that offers a nice set of geometric functions.To include this library into your build path you simply add the JTS Maven dependency to the pom.xml file in your projects root directory.
In addition Im using the GSON library to handle JSON within the Java backend. You can basically use any JSON library you want to. If you want to use GSON import this dependency.
Writing your Endpoints
Adding Fences to Cloud Datastore
For the sake of convenience youre only storing the fences vertices and some metadata. To send and receive data through the endpoints you need an object model which you need to create in a little Java Bean called MyFence.java.Now you need to create an endpoint called add. This endpoint expects a string for the group name, a boolean indicating whether to rebuild the spatial index, and a JSON object representing the fences object model. From this App Engine creates a new fence and writes it to Cloud Datastore.
Retrieving a List of our Fences
For some use cases it makes sense to fetch all the fences at once in the beginning, therefore you want to have an endpoint to list all fences from a certain group.Cloud Datastore uses internal indexes to speed up queries. If you deploy the API directly to App Engine youre probably going to get an error message, saying that the Datastore query youre using needs an index. The App Engine Development server can auto-generate the indexes, therefore Id recommend testing all your endpoints on the development server before pushing it to App Engine.
Getting a Fences Metadata by its ID
When querying the fences you only return the ids of the fences in the result, therefore you need an endpoint to retrieve the metadata that corresponds to a fences id.Building the Spatial Index
To speed up the geofencing queries you put the fences in an STR tree. The JTS library does most of the heavy lifting here, so you only need to fetch all your fences from the Datastore, create a polygon object for each one and add the polygons bounding box to the index.You then build the index and write it to memcache for fast read access.
Testing a point
You want to have an endpoint to test any latitude-longitude-pair against all your fences. This is the actual geofencing part. Thats so you will be able to know, if the point falls into any of your fences and if so, it should return the ids of the fences the point is in.For this you first need to retrieve the index from memcache. Then query the index with the bounding box of the point which returns a list of polygons. Since the index only tests against the bounding boxes of the polygons, you need to iterate through the list and test if the point actually lies within the polygon.
Querying for a Polylines or Polygons
The process of testing for a point can easily be adapted to test the fences against polylines and polygons. In the case of polylines you query the index with the polylines bounding box and then test if the polyline actually crosses the returned fences.When testing for a polygon you want to get back all fences that are either completely or partly contained in the polygon. Therefore you test if the returned fences are within the polygon or are not disjoint. For some use cases you only want to return fences that are completely contained within the polygon. In that case you want to delete the not disjoint test in the if clause.
Testing & Deploying to App Engine
To test or deploy your API simply follow the steps in the Using Apache Maven tutorial.Scalability & Pricing
The beauty of this is, since its running on App Engine, Googles platform as a service offering, it scales automatically and you only pay for what you use.If you want to insure best performance and great scalability you should consider to switch from the free and shared memcache to a dedicated memcache for your application. This guarantees enough capacity for your spatial index and therefore ensures enough space even for a large amount of complex fences.
Thats it - thats all you need to create a fast and scalable geofencing API.
Preview: Processing Big Spatial Data in the Cloud with Dataflow
In my next post I will show you how I geofenced more than 340 million NYC Taxi locations in the cloud using Googles new Big Data tool called Cloud Dataflow.
Smart Booster Pro Working v3 94 Apk Android
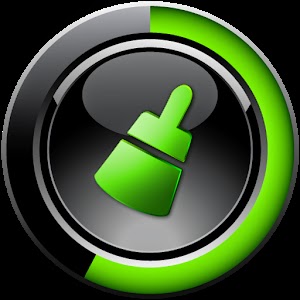
Smart RAM Booster: Small widget to adaptive boost RAM from anywhere
Fast cache cleaner: One click to clean cache
Quick SD card cleaner: Effectively scan and clean junk created by million apps
Advanced application manager: Optimize your devices by hibernate, disable, auto start apps
Key Features:-
- 1 click to clean cache
- Show detail internal cache and external cache
- Select apps that used the most cache
- Show SD card partition
- Scan for junk folder, big files, orphaned files
- Nice photo cleaner, music cleaner
- Notify for low storage usage
- Easily backup, uninstall unused apps
- App2SD Recommend app to move to SD card
- Disable system apps
- Scan auto start apps
- Manage backup (.apk) files.
Download APK:
click here
Google Play Store:
click here
Google Cast Remote Display Plugin for Unity
Posted by Leon Nicholls, Developer Programs Engineer
Today we launched the Google Cast Remote Display plugin for Unity to make it easy to take your Unity games to TVs. The Google Cast Remote Display APIs use the powerful GPUs, CPUs and sensors of your Android or iOS mobile device to render a local display on your mobile device and a remote display on your TV.
Unity is a very popular cross-platform game development platform that supports mobile devices. By combining the Google Cast Remote Display technology with the amazing rendering engine of Unity, you can now develop high-end gaming experiences that users can play on an inexpensive Chromecast and other Google Cast devices.

Games Using the Plugin
We have exciting gaming apps from our partners that already use the Remote Display plugin for Unity, with many more coming shortly.
Monopoly Here & Now is the latest twist on the classic Monopoly game. Travel the world visiting some of the worlds most iconic cities and landmarks and race to be the first player to fill your passport with stamps to win! Its a fun new way to play for the whole family.
Risk brings the original game of strategic conquest to the big screen. Challenge your friends, build your army, and conquer the world! The game includes the classic world map as well as two additional themed maps.
These games show that you can create games that look beautiful, using the power of a phone or tablet and send that amazing world to the TV.
Add the Remote Display Plugin to Your Game
You can download the Remote Display plugin for Unity from either GitHub or the Unity Asset Store. If you have an existing Unity game, simply import the Remote Display package and add the CastRemoteDisplayManager prefab to your scene. Next, set up cameras for the local and remote displays and configure them with the CastRemoteDisplayManager.
To display a Cast button in the UI so the user can select a Google Cast device, add the CastDefaultUI prefab to your scene.
Now you are ready to build and run the app. Once you connect to a Cast device you will see the remote camera view on the TV.
You have to consider how you will adapt your game interactions to support a multi-screen user experience. You can use the mobile device sensors to create abstract controls which interact with actions on the screen via motion or touch. Or you can create virtual controls where the player touches something on the device to control something else on the screen.
For visual design it is important not to fatigue the player by making them constantly look up and down. The Google Cast UX team has developed the Google Cast Games UX Guidelines to explain how to make the user experience of cast-enabled games consistent and predictable.
Developer Resources
Learn more about Googles official Unity plugins here. To see a more detailed description on how to use the Remote Display plugin for Unity, read our developer documentation and try our codelab. We have also published a sample Unity game on the Unity Asset Store and GitHub that is UX compliant. Join our G+ community to share your Google Cast developer experience.
With over 20 million Chromecast devices sold, the opportunity for developers is huge, and its simple to add Remote Display to an existing Unity game. Now you can take your game to the biggest screen in millions of users homes.
Saturday, September 17, 2016
Tell us about your experience building on Google and raise money for educational organizations!
Thats why were launching our developer survey -- we want to hear about how you are using our APIs and platforms, and what your experience is using our developer products and services. Well use your responses to identify how we can support you better in your development efforts.

The survey should only take 10 to 15 minutes of your time, and in addition to helping us improve our products, you can also help raise money to educate children around the globe. For every developer who completes the survey, we will donate $10 USD (up to a maximum amount of $20,000USD total) to your choice of one of these six education-focused organizations: Khan Academy, World Fund, Donors Choose, Girl Rising, Raspberry Pi, and Agastya.
The survey is live now and will be live until 11:59PM Pacific Time on October 15, 2014. We are excited to hear what you have to tell us!
Posted by Neel Kshetramade, Program Manager, Developer Platform